Exporting Debug Information¶
The NxLib can export debug information into a .enslog
file, which contains data in a proprietary binary format. These files can be visualized using the NxProfiler application. The .nxlog
extension is also supported to ensure backward compability.
Exporting Debug Information Using NxTreeEdit¶
You can use NxTreeEdit to connect to your application and export debug information.
In your application use nxLibOpenTcpPort to start a server to which NxTreeEdit can connect.
Open NxTreeEdit.
Connect to your application. Make sure to connect to the correct process. If e.g. NxView is running in the background it will also show up in the list of NxLib instances.
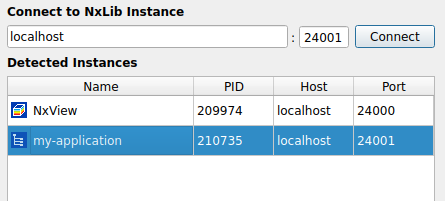
Open the debug tab at the bottom of the NxTreeEdit window.
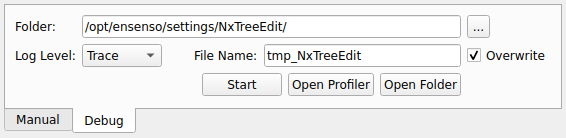
Select the debug level you want to use, which should be “Trace” for support inquiries.
Start the debugging.
Trigger the operations you want to observe in your application.
Stop the debugging and open or copy the resulting file.
Exporting Debug Information From Your Application¶
Debug Levels¶
Set the /Debug/Level node to enable logging. The available levels are
|
Disables profiling outputs. |
|
Outputs only basic information like command executions. Can be used without measurable influence on the application performance. |
|
Outputs more information about sub steps of commands or helper threads. |
|
Full logging including all API calls and parameter values. We recommend to use this level only for debugging purposes. |
NxLibItem()[itmDebug][itmLevel] = valTrace;
Enable Logging from the Start¶
Logging can be enabled even before the initialization of the NxLib by setting the environment variable NXLIB_PROFILE_LEVEL
to one of the levels above. The data can be retrieved as usual from NxTreeEdit or using the API.
Retrieving Debug Information¶
Retrieve the debug information using nxLibGetDebugBuffer and write it to a binary file with the extension .enslog
. Additional data from the next call to nxLibGetDebugBuffer can be appended to the same file.
Note
You have to retrieve debug information regularly. Otherwise the internal buffers will overflow and discard the oldest information that was not retrieved yet. nxLibGetDebugBuffer has an output parameter to indicate whether data was discarded.
std::vector<char> dbgBuffer;
bool bufferOverflow;
nxLibGetDebugBuffer(dbgBuffer, true, &bufferOverflow);
std::ofstream debugFile("debug.enslog", std::ios::out | std::ios::binary);
if (!dbgBuffer.empty()) {
debugFile.write(dbgBuffer.data(), dbgBuffer.size() * sizeof(dbgBuffer[0]));
}
set_framegrabber_param(Handle, 'do_export_debug_buffer', ['append', 'C:/path/to/file.enslog'])
Automatic Export with File Rotation¶
The NxLib can write debug information to files automatically in the background. This functionality can be enabled with the /Debug/FileOutput node. When starting the logging or after the current log file has reached MaxFileSize a new log file will be created automatically based on FolderPath and FilePrefix. Old log files will be deleted if the size of old logs with the same prefix exceeds MaxTotalSize.
NxLibItem debugOut = NxLibItem()[itmDebug][itmFileOutput];
// Set output directory
debugOut[itmFolderPath] = "C:\\my_log_folder";
// Keep 100MB of logging data
debugOut[itmMaxTotalSize] = 100e6;
// enable automatic writing of log files
debugOut[itmEnabled] = true;
Generate Custom Debug Information¶
An application can insert its own debug information into the NxLib’s internal logging mechanism. This allows to easily debug multithreaded timings and interactions between NxLib and application specific code in NxProfiler.
// The scope will determine the lifetime of the following NxLibDebugBlocks
{
// To track a certain function or code segment simply create a NxLibDebugBlock object
// The lifespan of this DebugBlock will be visible in the NxProfiler
NxLibDebugBlock db1("Example Block 1", nxdDebug);
// Simple message prints will inherit the debug level of its parent block
nxLibWriteDebugMessage("Example Message");
// do stuff...
// It's possible to nest the blocks into each other:
NxLibDebugBlock db2("Example Block 2");
// do more stuff...
// db1 and db2 will be destroyed here and the blocks will be implicitly closed
}